Express.js is a web application framework for Node.js. It provides various features that make web application development fast and easy which otherwise takes more time using only Node.js.
Express.js is based on the Node.js middleware module called connect which in turn uses http module. So, any middleware which is based on connect will also work with Express.js.
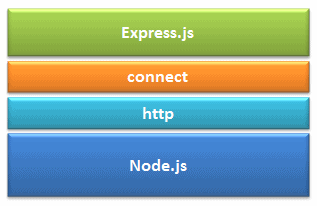
Advantages of Express.js
- Makes Node.js web application development fast and easy.
- Easy to configure and customize.
- Allows you to define routes of your application based on HTTP methods and URLs.
- Includes various middleware modules which you can use to perform additional tasks on request and response.
- Easy to integrate with different template engines like Jade, Vash, EJS etc.
- Allows you to define an error handling middleware.
- Easy to serve static files and resources of your application.
- Allows you to create REST API server.
- Easy to connect with databases such as MongoDB, Redis, MySQL
Install Express.js
You can install express.js using npm. For our purposes, the best way to set this up is using a VM Template that already exists in XOA.
Once your VM is up and running, login via SSH, change to root user and run the following command:
npm install -g express
The output should look like this:
administrator@ubuntu:~$ sudo -i [sudo] password for administrator: root@ubuntu:~# npm install -g express + express@4.17.1 added 50 packages from 37 contributors in 3.386s root@ubuntu:~#
Now, you are ready to use Express.js in your application.
Use Express.js
You can create a web application using Express.js by following this previous tutorial here.
Note that in the tutorial we define routes, but those are static or “fixed”. To use dynamic routes, we really SHOULD provide different types of routes. Using dynamic routes allows us to pass parameters and process based on them, which is much more flexible.
Here is an example of a dynamic route:
ar express = require('express'); var app = express(); app.get('/:id', function(req, res){ res.send('The id you specified is ' + req.params.id); }); app.listen(5000);
Visiting the new URL on the correct port of your VM (eg. http://10.108.155.143:5000/123) will output the following in the browser:
The is you specified is 123
You can replace ‘123’ in the URL with anything else and the change will reflect in the response. A more complex example of the above is:
var express = require('express'); var app = express(); app.get('/things/:name/:id', function(req, res) { res.send('id: ' + req.params.id + ' and name: ' + req.params.name); }); app.listen(5000);
You can use the req.params object to access all the parameters you pass in the url. Note that the above 2 are different paths. They will never overlap. Also if you want to execute code when you get ‘/things’ then you need to define it separately.